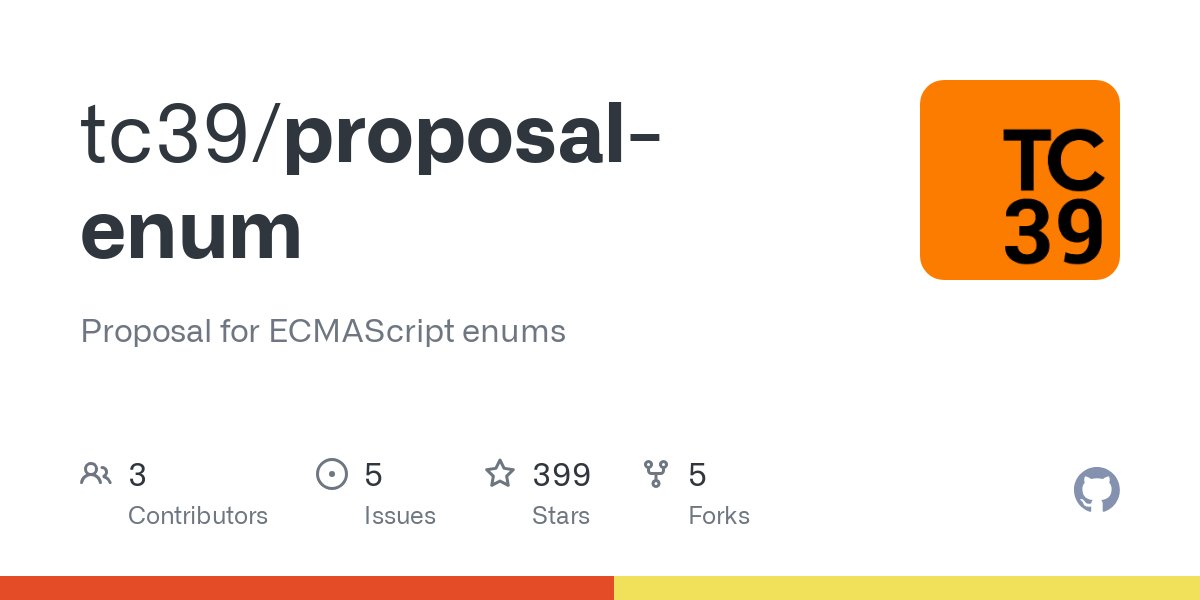
Enums in JS advancing to stage 1
4/16/2025
Enums are a part of many languages, but not yet a part of JavaScript.
They are useful to define a list of named constants. They usually have incremental values, for integer type, but can be assigned to any arbitrary value of the same type.
Let’s have an example with TypeScript:
enum Direction {
Up = 1,
Down,
Left,
Right,
}
The enum Direction defines 4 constants, starting with UP = 1. If we did not assign a value to UP, it would be 0.
Each following constant is auto incremented, this means Down === 2, Left === 3, etc.
So, for instance, you could use it with an hypothetical character class:
const mainCharacter = new Character();
mainCharacter.move(Direction.Right);
This is a more practical way than defining 4 constants (and importing 4 constants where you want to use them). The built-in autoincrement is also nice to have.
This is also a way to semantically group your constants and avoid name clashes: Up, Down, Left, Right could also be used to represent keyboard keys with a Key
enum.
Unfortunately Javascript does not have yet a standard to use enums, so each developer may implement enums-like in different ways, usually with an Object Literal.
Current proposal
For now, enums are a proposal of the TC39 working group, the people working on ECMAScript specification that JS engines should implement.
This proposal just advanced to stage 1.
The current proposal has some key differences with TypeScript enums:
- No default auto-initializers
- No declaration merging (a good thing if you ask me)
- No reverse-mapping (get an enum member name from a value)
- Allow more types to be assigned to enum members: Symbol, BigInt and Boolean values
In my opinion, auto-initializers should be a part of the standard, because sometimes all you need is the meaning of an enum member, and not the actual value.
The future
Opt-in auto-initializers
Opt-in auto-initializers are considered in the future of the proposal. 2 syntaxes are illustrated:
// The "of" clause, which can deal with multiple types
enum Numbers of Number { zero, one, two }
enum Colors of String { red, green, blue }
Numbers.zero; // 0
Colors.red; // "red"
// The auto keyword, only for numbers
auto enum Numbers { zero, one, two }
Pattern-matching
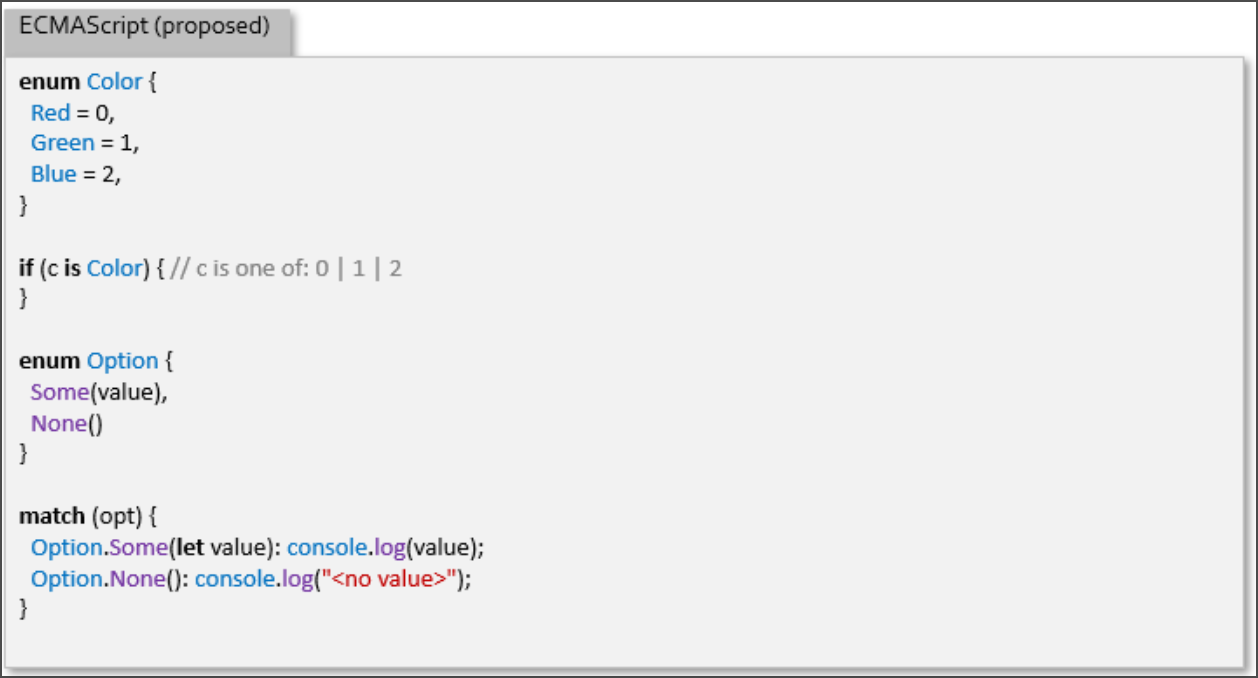
Decorators
Useful to serialize/deserialize enums.
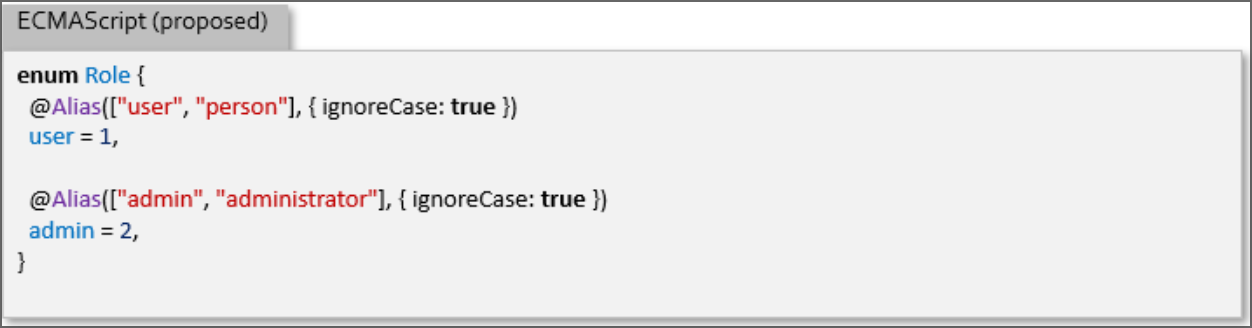
To summarize, good news for JavaScript as enums are advancing to the next step.
This standard way to define and use enums will make collaborative work easier, providing more info to the developer with static analysis than a simple Object Literal.